I recently had a discussion with a friend about the Model-View-ViewModel pattern (MVVM) for UI apps and the fact that it allows unit testing where you would not have thought it possible in the first place, for example, logic involved by drag and drop. We agreed on the fact that most of MVVM posts are very theoretical regarding MVVM and when they are not, the testing part, is only mentioned never detailed. That is why I decided to write The objective of this post is to detail the code architecture and the techniques involved to the testing of a very simple WPF app. This app enables the user to rank via drag and drop the list of the french football clubs and save this ranking. This could be a part of larger app that could be used, for example, to bet the final table… However for the sake of simplicity of this post we will focus mainly on the WPF control ListView containing the football club rows. The source code can be found in this github repository.
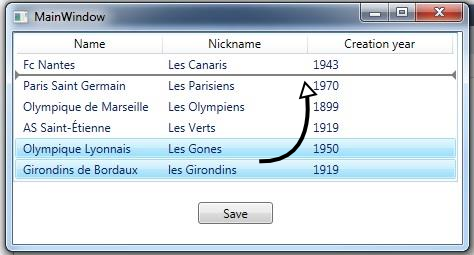
We will use an external library that wraps all the complex events handling regarding the mouse action involved in the drag and drop. Following unit testing principles, we will only test our logic which will be the movement of elements in the collection the ListView is bound to. In order to add little bit of extra complexity, we would like to allow not only the movement of one row but also of a block of contiguous rows.
There are tons of blog posts and articles on theoretical description of MVVM written by brilliant developers so I will try to be as brief as possible and will focus more on the example. The fundamental and natural principle on which MVVM and other UI pattern are based on is to separate the UI from the business logic. In one sentence, the application logic should not be tied to UI elements. The Model/View/ViewModel comes in three parts, it is well summarized in this blog, quoting:
A view is simply a UI page: It does not know where the data is coming from.
A ViewModel holds a certain shape of data, and commands, that a View binds to.
The model refers to the actual business data, which is used to populate the ViewModels.
The basic interaction rules can be summarized in the drawing below.
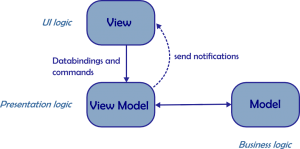
Speaking more in terms of WPF, the view contains the UserControls, the windows. A view class definition is split between the xaml file (simplifying UI design) and the associate .cs file called code behind. If an application is coded following MVVM principles the code behind should be small, containing code on the UI elements that are difficult to expressed with XAML syntax (e.g. keyboard bindings).
Now it is time, to present the external tools that we are going to use in this small app. The drag and drop mouse interaction will be handled by the open source project GongWPF. For unit testing we will use the Visual Studio Test framework and Moq for creating mock objects. For a real and more sophisticated app written with MVVM, I would recommend you to use a framework. Personally, I do like MVVMLight.
Let us describe the code of our app starting by the so-called model part. This is a natural way because, in real scenarios this may be existing parts, written before ever considering the UI. However, in this fake app it is going to be very simple. The main business interface is IFootballClub exposing three properties regarding the club.
The core model is represented by the interface IChampionship. It is extremely lightweight in our situation because, in this post, we want to focus more on the View/ViewModel interaction. However, we have added the property CurrentChampionShipRanking and GetGoodBetCount as examples of methods that could be put in the model.
Let us now present the interface for our ViewModel, IChampionshipViewModel, which is the most important part of this blog post. Therefore, it will be the only interface where we provide the implementation. This implementation will handle the drag and drop core logic and will be the system under test for our matter. The list of football clubs will be kept in the FootballClubs observable collection. This collection handles natively all the notifications for the view. The Save action will be handled by the SaveClick property whose type is ICommand. An ICommand is an interface for an action that can be executed.
Remark also that IChampionshipViewModel extends IDropTarget which is the main interface provided by GongWPF for handling the drag and drop events. This interface contains two methods void DragOver(IDropInfo dropInfo) and void Drop(IDropInfo dropInfo)
To complete the overview let us present the xaml for our view. Indeed, following MVVM principles, our custom UserControl is essentially XAML leaving no code behind. The most important part is the binding of the View to the ViewModel via the DataContext property. In our case, we have used a ViewModelLocator (a very simple one with no IoC container) see this post for more information on the ViewModelLocator pattern. Naturally, the ListView ItemsSource (the list of rows) is bound to the FootballClubs property of the IChampionshipViewModel interface. For each column, we can bind to a property of the interface IFootballClub. Note also, that the Resharper AddIn enables intellisense and type validation of those bindings which is really valuable for early detection of errors.
Finally, the ListView control has the following GongWPF attributes:
dd:DragDrop.IsDragSource=”True”, dd:DragDrop.IsDropTarget=”True”, dd:DragDrop.DropHandler=”{Binding}”
Thanks to these attributes, GongWPF will be able to make a bridge between the mouse UI events and the methods of the IDropTarget interface which our ViewModel extends.
Unfortunately, as brilliant as it is, GongWPF does not do everything and it’s our responsibility to implement the logic that moves the elements within the ObservableCollection FootballClubs.
Following the TDD principles let us write tests first. But before that, let us have a glimpse at our SUT (system under test) which is the class ChampionshipBetViewModel. The model IChampionship is injected as a constructor parameter. We will not discuss further the SaveClick part but we use a custom implementation of the ICommand interface which basically execute the lambda expression passed as parameter. This Action typed lambda updates our model with the reranked list of football clubs.
Here comes our first unit test. I think it is a good thing when a test can expressed in terms of a simple sentence such as “Given… When … Then…”. Logically, the test method name should be closed to this sentence. The first one will assert that “ given a list of four rows when the the source is the first row and the target is the second one then the list of row should be reordered”. Remark that the target (the InsertIndex in GongWPF API) corresponds to the row instance preceding the inserted item. Being clear with this convention, the GongWPF interfaces are easily mocked using Moq, resulting in the following unit test. We assert that after executing the Drop method the list is club2, club1, club3, club4
For having a proper case coverage it is also important to assert "negative" situations such as the following one "given a list of four rows, if the source is the block containing the two last rows and the target is the second one then the drop action should not change the order"
Remind, that we have for specification to be able to move a block of contiguous rows at once but we do not want to move two discontinuous blocks. Therefore, the DragOver method should handle properly this situation: the styling that allows insertion should be set in the first situation and not set at all in the second one. To assert this with unit tests, we use the VerifySet methods on the mock object dropInfo. For both properties DropTargetAdorner and Effects, we verify they are set when the selected items forms a contiguous block and not set when the two selected rows are not adjacent. If those conditions are not met the tests would fail, indeed, Moq framework would throw exceptions.
We finish this post by showing the true implementation of the ViewModel. We do not provide the details of the method MoveAllLeft and MoveAllRight they can be found on the github (they probably can be reviewed and shortened).
To conclude, we have shown the basic ingredients for the testing of a ViewModel, this tests suite can be extended to complete a strong code coverage (there are many corner cases in our situation). Not also, that the MVVM pattern allows you to test the command, for example the SaveClick. Thanks to the Moq VerifySet method, you can check that the setter on the UserBet property for the mock Mock
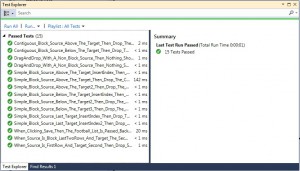