In this post I describe the tools that I have selected for efficient development of javascript tests within Visual Studio. Indeed, if you are developing sites with ASP.NET or Apps for Office then you are more or less committed to use Visual Studio. Therefore, you probably do not want to use another editor for your javascript development.
What were my requirements when I chose the tools that I will describe to you in this post? Actually, I needed tools which can provide me the same comfort that I have while I am developing in TDD with .NET. Precisely, the test should be run individually (or at least individually for a given set). They should be run on the continuous integration build, which is teamcity in my case. The test runner may use any browser for executing the tests even if flexibility for using all kind of browsers would be appreciated. In addition, the test should be easily debugged and this is a very important aspect for me.
Let me detail a little this latter requirement. Indeed, I have read recently this blog post and its written that
Debugging the tests
Wiseman said: “If you need to debug unit test then something is wrong with it’s unitness.”
PS: If it’s really needed you can debug it in browser as you normally debug JavaScript code.
While if the rest of the blog is brilliant, I do not subscribe at all to this sentence. If you practice regularly TDD then you’ll see that you have to debug, that’s part of the game. If you never have to debug maybe it’s because you are asserting too much trivialities and not enough your own complex logic. In addition, if you have to create the webpage on your own, for debugging, that is not acceptable to me either. You should be able to put your breakpoint anywhere in your code and hit it in less than five seconds. We will see that the tool chosen permits such quick debugging and that’s the main reason why I am using it.
At the time of the writing, the popular Visual Studio Addin Resharper with its version 8 supports javascript tests with the frameworks Jasmine or QUnit. Unfortunately, the debugger cannot be properly attached while debugging the scripts. Sadly, I had to reject Resharper that I appreciate so much for .NET development.
Finally, the best tool that I found is Chutzpah (which means Audacity in Yeddish). Firstly, there is the Chutzpah runner which is a javascript test runner that uses the headless browser phantomJs, we will invoke it from the command line in the continuous build. Sedondly, there is the Chutzpah Visual Studio extension which enables quick runs and debugging sessions from Visual Studio. Both runner and Visual Studio extension is fully compatible with JS test framework Jasmine 2.0. that I have chosen. Note that I also use the mocking library sinonJs but I won’t discuss it there.
You may retrieve the code samples below on my github repository.
To illustrate these tools we will use a very simple mockup project and we start its description with the following VisualStudio solution.
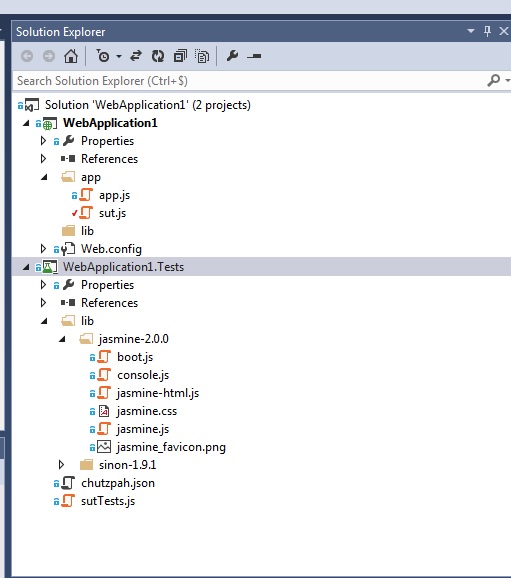
As usual there are two projects: WebApplication1, the core project, containing the app folder with our javascript logic, especially the one that we would like to test: sut.js (for SystemUnderTest). There is also the test project, WebApplication1.Tests. The file testing the logic contained in sut.js is simply sutTests.js. The testing framework Jasmine is added to the solution by referencing the folder lib/jasmine-2.0.0..
We will take a very simple example, where the object app.sut has a function for computing the factorial of an integer. The code of sut.js can be found in the following snippet.
(Note that in app we have implemented a basic namespacing function such as this one)
Let us now focus on the test suite in the sutTests.js code.
This piece of code is very basic Jasmine syntax for a test suite. We assert the values of the factorial function for the two corner cases where the input is 0 and 1 and we also test the situation with n=5. Remark also that we have a test which asserts that an exception is thrown when a non positive value is passed to the function. We have not covered this situation with the snippet above, consequently, we expect this test to fail… Remark that all the needed references to js files are handled with ///<reference of Visual Studio. By the way, we benefit from Visual Studio Intellisense here.
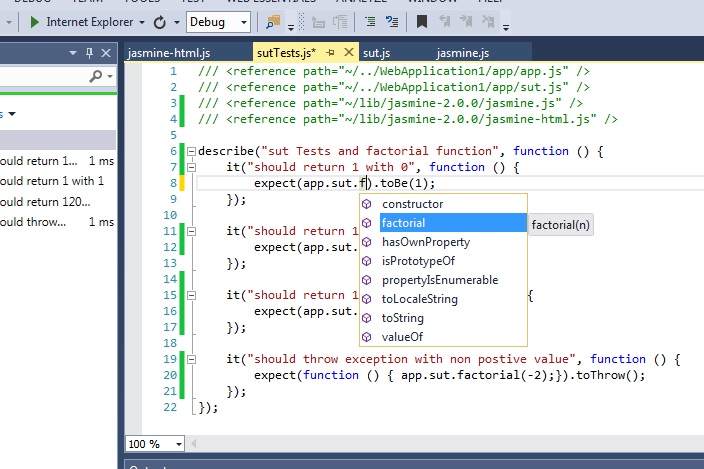
In the next screen shot, we run the tests with the Visual Studio Test Explorer that is compatible with Jasmine thanks to Chutzpah.
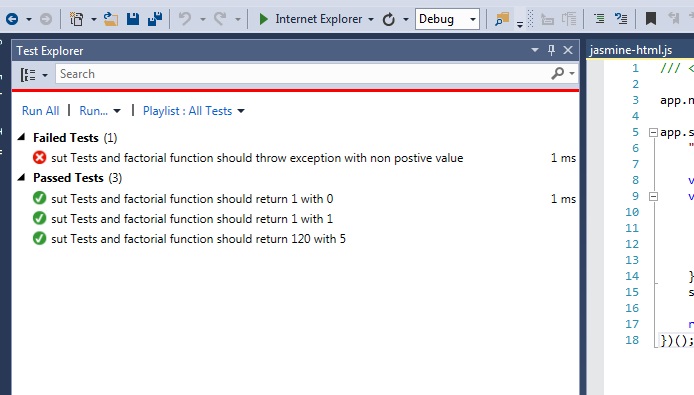
If we were in the situation where we do not know what is going wrong (which is often the case) we would need to debug the test. Unfortunately, like Resharper, you cannot debug the test directly in VisualStudio, the debugger does not attach well. Even Chutzpah creator does not know how we would do that, so I believe we will have to wait to debug javascript test as easily as we debug .NET within visual studio. Then, we will have to debug with a web browser. However, Chutzpah creates the webpage for bootstrapping your failing test. So just by clicking in Open in browser you will have the web page loaded and a link to run the failing test within the browser (see screenshots below).
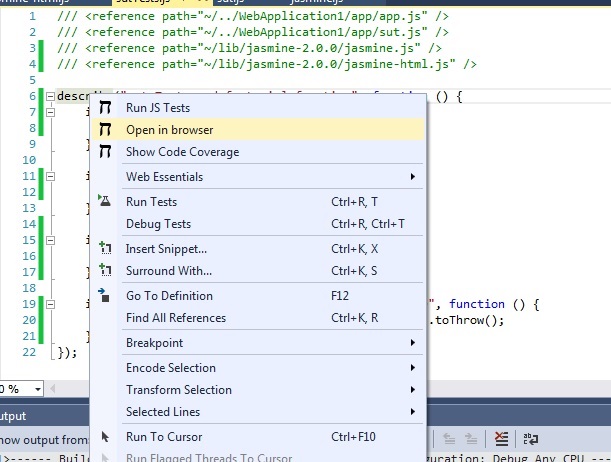
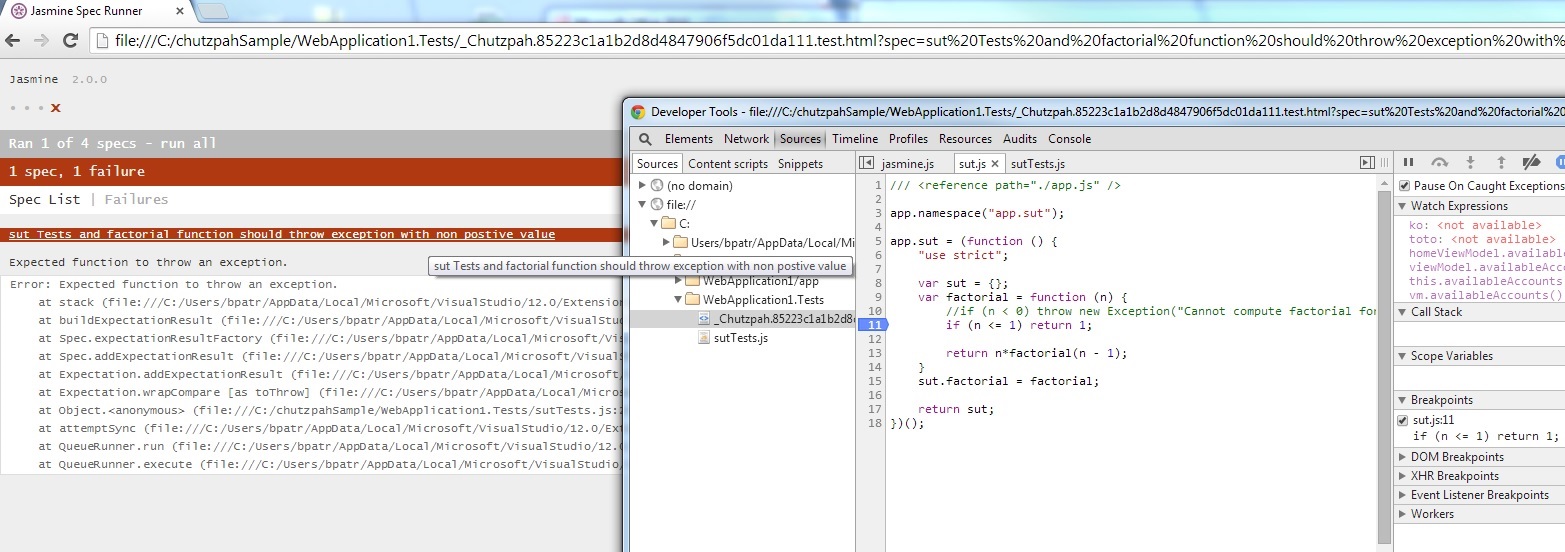
Now, we do have all the material for editing efficiently tests in Visual Studio. Let us have a few words for running the test using the command line on the server. Personally, I embed the Chutzaph runner in a /build directory within my sources and executes the following Powershell script.
Here is what it looks when ran it an Powershell console with teamcity options.
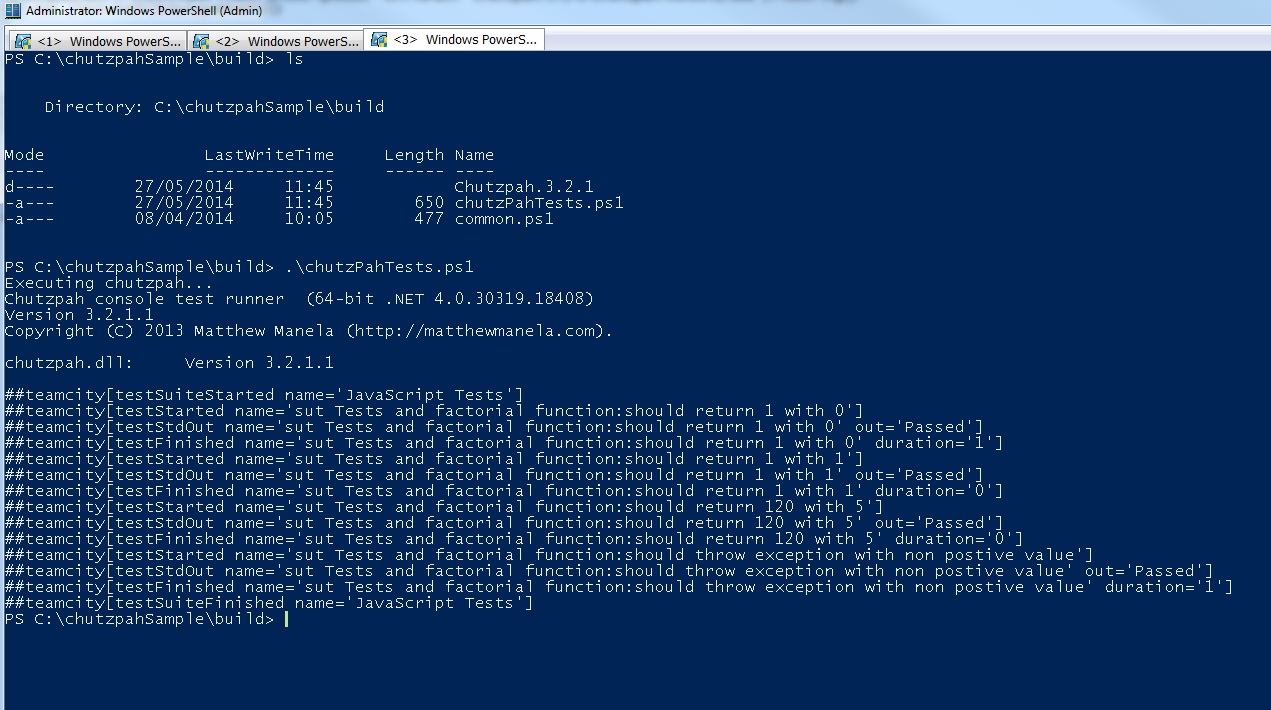
To conclude, I would say that Chutzpah is a great project and if you need a simple and ready to use test runner I would recommend it. The only limitation for now would be that the runner only supports phantomJS. However, you may use another another test runner for executing the tests with different browser and you can keep the Chutzpah Visual Studio extension for the development. One last important thing to note is the fact that Chutzpah 3.0 supports RequireJs.